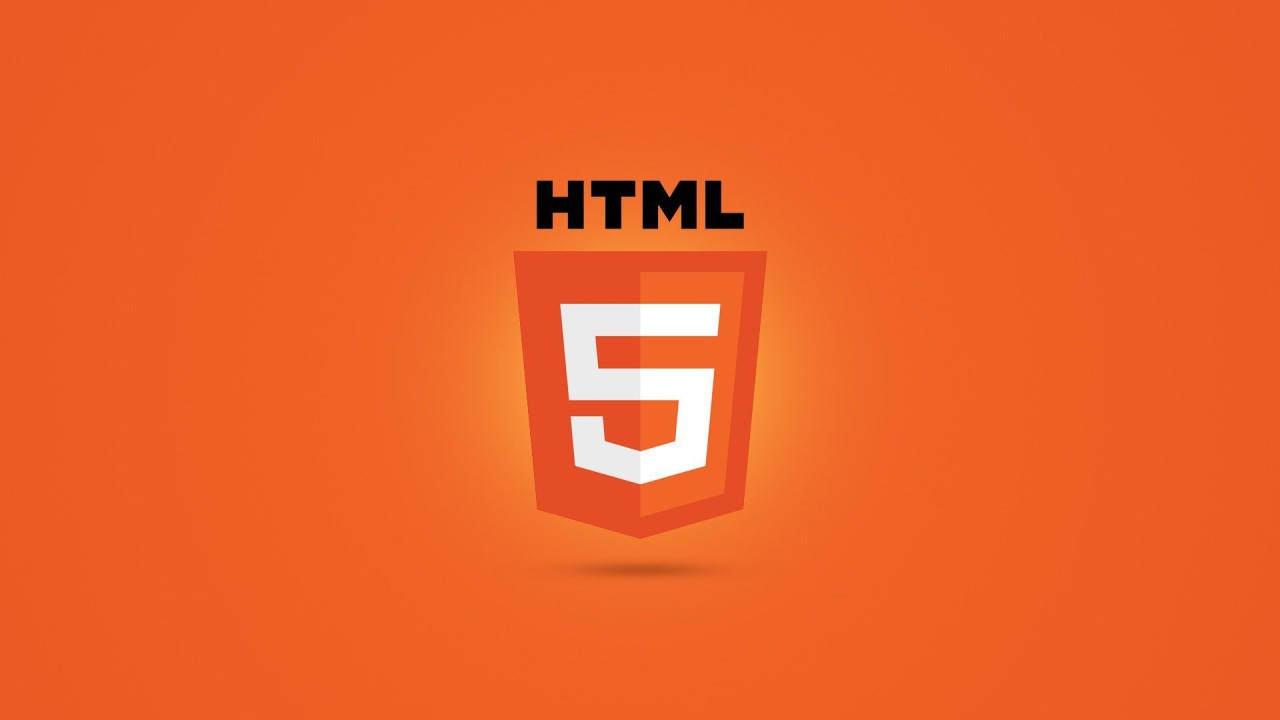
Introduction
Forms are an essential part of web development, allowing users to input and submit data. Whether it's a login page, a contact form, or a registration form, HTML provides various elements to build interactive forms efficiently. In this guide, we'll explore everything from basic form creation to advanced input types and validation techniques.
1. Creating Forms (<form>
)
The <form>
element is the container for all form elements. It collects user input and can send it to a server for processing.
<form action="submit.php" method="POST"> <!-- Form elements go here --> </form>
Key Attributes of <form>
:
action
: Specifies the URL where form data should be sent.method
: Defines the HTTP method (GET
orPOST
).autocomplete
: Enables or disables autofill for form fields.target
: Specifies where to display the response (_self
,_blank
, etc.).
2. Form Elements
HTML provides different elements for user input.
2.1 <input>
Element
The <input>
element is one of the most used form elements. It supports various types such as text, password, email, etc.
<input type="text" name="username" placeholder="Enter your name">
2.2 <textarea>
Element
Used for multi-line text input.
<textarea name="message" rows="4" cols="50">Type your message...</textarea>
2.3 <select>
Element
Dropdown menus allow users to select one or more options.
<select name="country"> <option value="us">United States</option> <option value="uk">United Kingdom</option> <option value="ca">Canada</option> </select>
2.4 <button>
Element
Defines a clickable button, often used for form submission.
<button type="submit">Submit</button>
3. Input Types
The type
attribute of <input>
allows defining various types of user input.
Input Type Description text
Single-line text input email
Email input with validation password
Password input (hidden characters) checkbox
Allows multiple selections radio
Allows selecting only one option per group file
Upload files number
Numeric input with min/max values date
Date picker color
Color picker range
Slider for selecting a range Example of radio buttons and checkboxes:
<label> <input type="radio" name="gender" value="male"> Male </label> <label> <input type="radio" name="gender" value="female"> Female </label> <label> <input type="checkbox" name="subscribe" value="yes"> Subscribe to Newsletter </label>
4. Labels and Fieldsets
Labels and fieldsets enhance form accessibility and organization.
4.1 <label>
Element
Associates text with a form control, improving accessibility.
<label for="email">Email:</label> <input type="email" id="email" name="email">
4.2 <fieldset>
and <legend>
Used to group related form elements together.
<fieldset> <legend>Personal Information</legend> <label for="fname">First Name:</label> <input type="text" id="fname" name="fname"> </fieldset>
5. Form Validation
HTML5 provides built-in form validation to ensure correct data submission.
5.1 Required Fields
The required
attribute ensures that a field is not left empty.
<input type="text" name="name" required>
5.2 Email Validation
Ensures input matches an email format.
<input type="email" name="email" required>
5.3 Pattern Matching
The pattern
attribute uses regex to enforce specific input formats.
<input type="text" name="zip" pattern="[0-9]{5}" title="Enter a 5-digit ZIP code">
5.4 Min and Max Values
Limits the range of numerical inputs.
<input type="number" name="age" min="18" max="60">
5.5 Custom Error Messages
The oninvalid
event allows setting custom messages.
<input type="text" name="username" required oninvalid="this.setCustomValidity('Username is required!')" oninput="this.setCustomValidity('')">
Conclusion
HTML forms are crucial for user interaction, enabling seamless data collection. By using different form elements, input types, labels, and validation techniques, you can create user-friendly and accessible web forms. Mastering these elements will improve both the user experience and data accuracy.
Leave a Comment